Read Email File
Here in this topic we have copied the Microsoft Email Message .eml file to some folder and now we need to read that file through code below is the code to read the file.
(1)Below is the code to read the .eml file subject body and from:-
const { simpleParser } = require('mailparser');
const fs = require('fs');
// Function to process .eml file
async function processEmlFile(filePath) {
try {
// Read the .eml file content
const rawEmailContent = fs.readFileSync(filePath, 'utf-8');
// Parse the raw email content
const parsed = await simpleParser(rawEmailContent);
// Extract the subject and body
console.log('Subject:', parsed.subject);
console.log('Body (Text):', parsed.text); // For plain text body
console.log('Body (HTML):', parsed.html); // For HTML body
// Extract and save attachments (if any)
if (parsed.attachments.length > 0) {
parsed.attachments.forEach((attachment) => {
console.log('Attachment:', attachment.filename);
// Save the attachment to the local filesystem
fs.writeFileSync(`./${attachment.filename}`, attachment.content);
console.log(`Attachment ${attachment.filename} saved.`);
});
}
} catch (error) {
console.error('Error parsing .eml file:', error);
}
}
// Example usage: Pass the path to the .eml file
processEmlFile('EmailFile.eml');
(2)In this code you will also get the text of the attachment file also:-
const fs = require('fs');
const path = require('path');
const { simpleParser } = require('mailparser');
// Function to read and extract attachment content from a .eml file
async function readAttachmentFromEML(emlFilePath) {
try {
// Read the .eml file
const emlData = fs.readFileSync(emlFilePath, 'utf-8');
// Parse the .eml data to extract email content and attachments
const parsedEmail = await simpleParser(emlData);
// Check if there are attachments in the email
if (parsedEmail.attachments && parsedEmail.attachments.length > 0) {
parsedEmail.attachments.forEach((attachment, index) => {
console.log(`Attachment ${index + 1}:`);
console.log('Filename:', attachment.filename);
console.log('Content Type:', attachment.contentType);
console.log('Content ID:', attachment.cid);
// Output the attachment content as base64
console.log('Content (base64):', attachment.content.toString());
// Optionally save the attachment to a file
const outputFilePath = path.join(__dirname, attachment.filename);
fs.writeFileSync(outputFilePath, attachment.content);
console.log(`Attachment saved as ${outputFilePath}`);
});
} else {
console.log('No attachments found.');
}
} catch (error) {
console.error('Error parsing .eml file:', error);
}
}
// Example usage: specify the path to your .eml file
readAttachmentFromEML('EmailFile.eml');
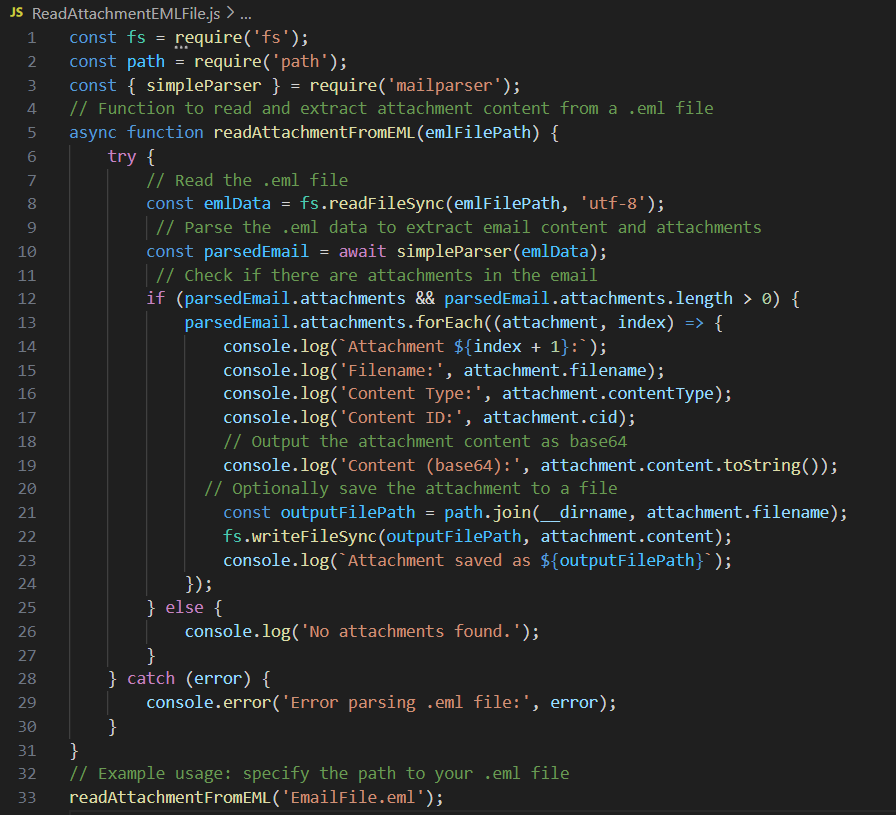 | |